CSS Center Image – How to Center an Image in CSS
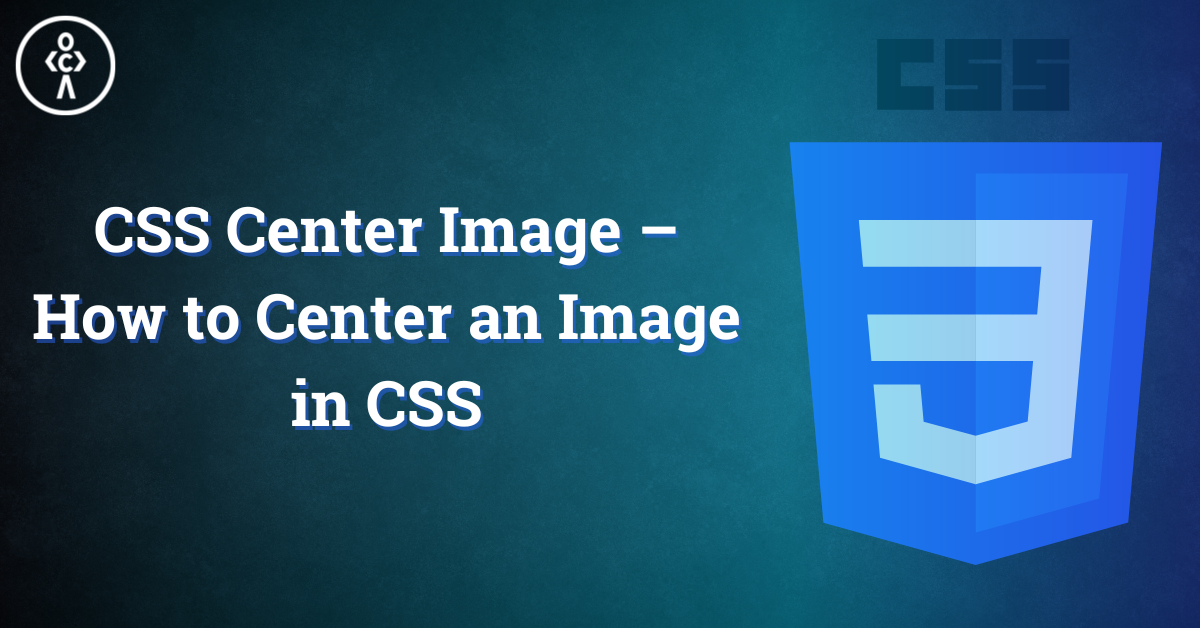
While building our website we often have to deal with images. A lot of time we have to align those images in CSS. To center an image using CSS is really popular frontend interview question as well because there are multiple ways to achieve it.
So, in this article, we will go through the various methods on how to center an image in CSS and understand each of them.
Before starting let’s set up our environment first.
Environment Setup
Using Codedamn Playground (Recommended)
The easiest way to set up the development environment would be to just use a codedamn playground. Using the playground you can see updates of the result in real-time in the preview section as you change the source code. It doesn’t need you to install anything on your machine.
Follow the steps to create your own codedamn playground.
- Go to Codedamn Playgrounds.
- Select the very first playground template “HTML/CSS”. It will ask you for the name of the project, you can type anything you want. And then, click on “Create Playground”.

- It should open an IDE in your browser.

In the leftmost section, we have our HTML, CSS, and JS files (we won’t need the JS file in this article). On the rightmost section, we have the preview section where we can see the changes in real time.
Local Setup
To setup your environment you can either use an editor like VS Code or Sublime Text to create HTML
and CSS
file.
And then open the file in any web browser like Chrome, Edge, etc. Remember, every time you change your source you have to reload the tab in the browser, otherwise, you have to use some 3rd party extensions like LiveServer of VS Code to automatically reload the website on changes.
With the setup done, let’s start with centering an image.
Adding an image
In the HTML file, remove the boilerplate code if you have any. And then add the following code.
<!doctype HTML>
<html>
<head>
<title>codedamn HTML Playground</title>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="/style.css" />
</head>
<body>
<h1>Centering an Image</h1>
<img src="https://picsum.photos/300/200" />
</body>
</html>
Code language: HTML, XML (xml)
Here we have added an image (<img>
) tag with a source attribute which is the URL of the image. We have used Lorem Picsum to get a random image. You can use any image you want. We have also linked the style.css
file to the HTML file using the <link>
tag
Now, let’s add some CSS to center the image.
Centering an image
Method 1: Using Flexbox
When it comes to aligning or arranging items, developers usually prefer to use CSS Flexbox. Flexbox is a very powerful tool for aligning items in a container. It is also very easy to use.
The important thing to note is that the flexbox is used to align items inside a container. This means we have to wrap the image inside a container and then use a flexbox on the container to center the image.
So, in the HTML file, add a div tag with a class name container
and wrap the image inside it.
<div class="container">
<img src="https://picsum.photos/300/200" />
</div>
Code language: HTML, XML (xml)
Everything still looks the same, because we haven’t added any CSS yet. Let’s add some CSS to center the image. Open the style.css
file and add the following CSS.
.container {
display: flex;
justify-content: center;
align-items: center;
}
Code language: CSS (css)
Whoa! We have centered the image using flexbox. Let’s understand what we have done.
- The
display: flex
property is used to make the container a flex container. - And then, we used the justify-content: center property to center the image. The justify-content property is used to align items along the main axis. The main axis is the horizontal axis in a flex container. So, the justify-content: center property will align the items horizontally in the center.
- And then, we used the align-items: center property to align the items in the center of the cross-axis. The cross axis is the vertical axis in a flex container. So, the align-items: center the property will align the items vertically in the center.

Method 2: Using Grid
CSS Grid is another powerful tool, very similar to that of flexbox. While flexbox really shines when it comes to one-directional flow, Grid was designed mainly for two-dimensional layouts. So, let’s see how we can use CSS Grid to center an image.
Very similar to flexbox, we have to wrap the image inside a container and then use CSS Grid to center the image.
Let’s copy the code from the previous section and paste it into the HTML file. And then, rename the class name of the div tag to container-2
.
<div class="container-2">
<img src="https://picsum.photos/300/200" />
</div>
Code language: HTML, XML (xml)
Now, let’s add some CSS.
.container-2 {
display: grid;
place-items: center;
}
Code language: CSS (css)
This should center the image. Let’s understand what we have done.
- The
display: grid
property is used to make the container a grid container. - The
place-items
property is actually responsible for both horizontal and vertical alignment. It is a shorthand property foralign-items
andjustify-items
.
So we could also write the CSS like this and achieve the same result.
.container-2 {
display: grid;
align-items: center;
justify-items: center;
}
Code language: CSS (css)

But we should always prefer the shorthand way.
Method 3: Using text-align (Horizontal Centering)
The text-align
property is used to set the horizontal alignment of all the inline content inside a container. So, we can use this property to center the image which is an inline element by itself.
Let’s name our container as container-3
and add the following CSS.
.container-3 {
text-align: center;
}
Code language: CSS (css)
It takes left|right|center|justify|initial|inherit
values only. In our case, we have used center
value to center the image.

Method 4: Using margin
We use the margin
property of CSS to create some space around the elements. If we can balance the space on all sides then it should enable us to center the image. For that, we can set the margin of the image to auto which will split the leftover space equally on all sides.
So, let’s name our container as container-4
and add the following CSS.
.container-4 {
margin: 0 auto;
}
Code language: CSS (css)
The 0
is for setting the margin value to zero on all sides. And then, we have set the margin to auto
which will split the leftover space.
Well, that doesn’t seem to work. Let’s add a border and see what has happened.
.container-4 {
margin: 0 auto;
border: 2px solid red;
}
Code language: CSS (css)

The problem is, that the wrapper div is taking up the entire horizontal space. So, we have to set the width of the wrapper div to the width of the image (its content). So, let’s add the width to the container.
.container-4 {
margin: 0 auto;
border: 2px solid red;
width: 300px;
}
Code language: CSS (css)
Now it should work.

Clearly, this is not a very good way to center an image, because we are changing the width of the wrapper container. Also, updating the image would require updating the CSS.
Method 5: Using margin contd.
So, let’s remove the container and try to center the image by itself.
Copy the image tag without the container and let’s add a class image-5
to it.
<img class="image-5" src="https://picsum.photos/300/200" />
Code language: HTML, XML (xml)
And then add this CSS:
.image-5 {
margin: 0 auto;
}
Code language: CSS (css)
Still, the image is not centered. Why? Because margin will work for block elements only. So, we have to make the image a block element. We can do that by adding the display: block
property to the image.
.image-5 {
display: block;
margin: 0 auto;
}
Code language: CSS (css)
And now it works !!

Method 6: Using position (Absolute Centering)
Using the position
property, we can position an element anywhere on the page. So, we can use this property to center the image as well.
Let’s name our new container with the image as container-6
and add the following CSS.
.container-6 {
position: aboslute;
left: 50%;
}
Code language: CSS (css)
What does this do?
The position: absolute
property positions the element relative to the nearest positioned ancestor. In our case, it will position container-6 relative to the document body cause that’s its ancestor.
The left
property determines the horizontal position of a positioned element. With left:50%
we are telling the browser to position the element 50% from the left of the document body.
This is a sketch of what it would look like:

Now, we have to move the image to the left by 50% of its width. But this has to be relative to its parent i.e container-6
. So, we have to use the transform
property to move the image to the left by 50% of its width.
.image-6 {
display: block;
transform: translateX(-50%);
}
Code language: CSS (css)

We can also use the left
property again to move the image to the left by 50% of its width.
.image-6 {
position: relative;
left: -50%;
/* transform: translateX(-50%); */
}
Code language: CSS (css)
NOTE: The reason we are using negative is because here the positioning is relative and we want to shift it left relative to the container.

Voila! We have successfully centered the image using the position
property.
Final Code
Here is the final HTML code with all the approaches.
<!doctype HTML>
<html>
<head>
<title>codedamn HTML Playground</title>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="/style.css" />
</head>
<body>
<h1>Centering an Image</h1>
<h2>Method-1: Using Flex</h2>
<div class="container">
<img src="https://picsum.photos/300/200" />
</div>
<br>
<h2>Method-2: Using Grid</h2>
<div class="container-2">
<img src="https://picsum.photos/300/200" />
</div>
<br>
<h2>Method-3: Using text-align</h2>
<div class="container-3">
<img src="https://picsum.photos/300/200" />
</div>
<br>
<h2>Method-4: Using margin</h2>
<div class="container-4">
<img src="https://picsum.photos/300/200" />
</div>
<br>
<h2>Method-5: Using margin without wrapper</h2>
<img class="image-5" src="https://picsum.photos/300/200" />
<br>
<h2>Method-6: Using position</h2>
<div class="container-6">
<img class="image-6" src="https://picsum.photos/300/200" />
</div>
</body>
</html>
Code language: HTML, XML (xml)
And the CSS file:
.container {
/* Method-1: Using Flex */
display: flex;
justify-content: center;
align-items: center;
}
.container-2 {
/* Method-2: Using Grid */
display: grid;
justify-items: center;
}
.container-3 {
/* Method-3: Using text-align */
text-align: center;
}
.container-4 {
/* Method-4: Using margin */
margin: 0 auto;
/* border: 2px solid red; */
width: 300px;
}
.image-5 {
/* Method-5: Using margin without wrapper */
display: block;
margin: 0 auto;
}
.container-6 {
/* Method-6: Using position */
position: absolute;
left: 50%;
}
.image-6 {
position: relative;
left: -50%;
/* transform: translateX(-50%); */
}
Code language: CSS (css)
You can also access my codedamn playground here.
References
Conclusion
In this article, we have seen how to center an image using different methods. We have seen how to use flexbox, grid, text-align, margin, and position to center an image.
I hope you enjoyed the article. Feel free to connect with me and follow me @ArnabSen1729.
Sharing is caring
Did you like what Arnab Sen wrote? Thank them for their work by sharing it on social media.
No comments so far
Curious about this topic? Continue your journey with these coding courses:


