How to Reverse a String in JavaScript

Strings are one of the most important parts of JavaScript and it’s useful for holding data in text form. Reversing a string is one of the most important technical questions asked in JavaScript interviews. Doing it with the right approach is very important.
Introduction
String is a sequence of characters that is used to hold and manipulate characters.
In javascript, there are various methods and functions available to manipulate string data like:
length()
: Used to calculate string length.slice()
: Extracts part of the string and returns extracted string in a new string.concat():
It is used to join two different stringsreplace():
It is used to replace the content of the stringtoUpperCase():
It is used to convert a string into uppercase.
There are other methods available in JavaScript to manipulate string data but there is no built-in function available to reverse a string. There are different ways to reverse a string but in this article, we will look at the 3 different and most interesting ways to do it.
Reverse a string with Array manipulation
Time needed: 5 minutes.
Reversing a string
- Split the string with split() function
Split string into individual characters
- Reverse the string with reverse() function
Reverse the array of characters with reverse() function
- Join the string with join() function
Finally join the reversed array with join() function
Let’s understand these steps in detail:
Step 1: split()
– This method splits the string into a separated array
let name = "codedamn";
const result = name.split(""); // outputs: ["c","o","d","e","d","a","m","n"]
Code language: JavaScript (javascript)
Step 2: reverse()
– reverse method reverses an array in a way that the first element becomes last and the last one becomes first but it only works on the array.
// it will return ["n", "m", "a","d","e","d","o","c"]
Code language: JavaScript (javascript)
Step 3: join()
– join method joins elements of an array into a single string.
We have to make a chain of these three methods to reverse the string and after chaining of three methods the code looks like
const str = "codedamn"
function reverseAString(str) {
return str.split("").reverse("").join("");
}
console.log(`Reversed string is: ${reverseAString(str)}`)
Code language: JavaScript (javascript)
The above code will return the following output
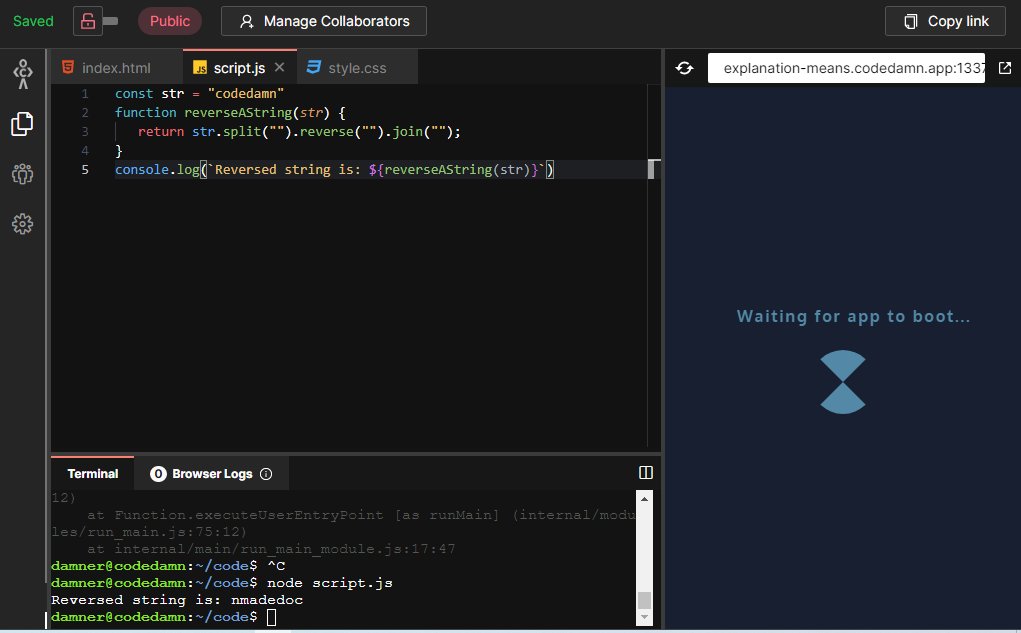
Reverse a string with a for-loop
We reversed a string using Javascript built-in functions. If there is a condition to not use built-in functions to reverse a string, then we have to find another way to reverse a string. That is where we can use a for-loop.
Let us achieve the same results by using a loop. We just need to apply suitable logic:
- Firstly, we are going to use a loop but we have to reverse the string.
- It means we use a loop in decrement order and we need the
length()
function to calculate string length. - We have already discussed
length
in the above section. The actual code looks like the below code:
function reverseString(str) {
let newString = "";
for (let i = str.length - 1; i >= 0; i--) {
newString += str[i];
}
return newString;
}
console.log(`Reversed string is : ${reverseString('codedamn')}`)
Code language: JavaScript (javascript)
The expected output for the above code will be:
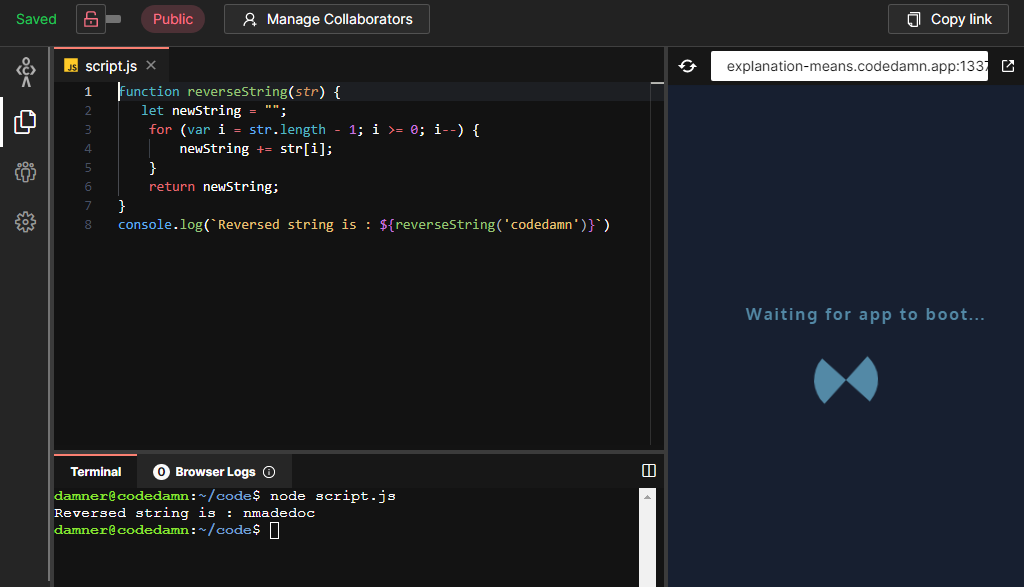
Reverse a string with the Recursion approach
This is another method to reverse a string. In programing competitions or technical interviews, you might have to solve the problem in a short and more readable form. We can achieve this with the help of recursion.
We are using two different methods to solve this problem
substr()
: It returns an extracted part of the string that begins at a specified location.charAt()
: It returns a specified character from the string
function reverseString(str) {
if (str === "")
return "";
else
return reverseString(str.substr(1)) + str.charAt(0);
}
console.log(`Reversed string is : ${reverseString('codedamn is awesome')}`)
Code language: JavaScript (javascript)
The above code will generate the following output
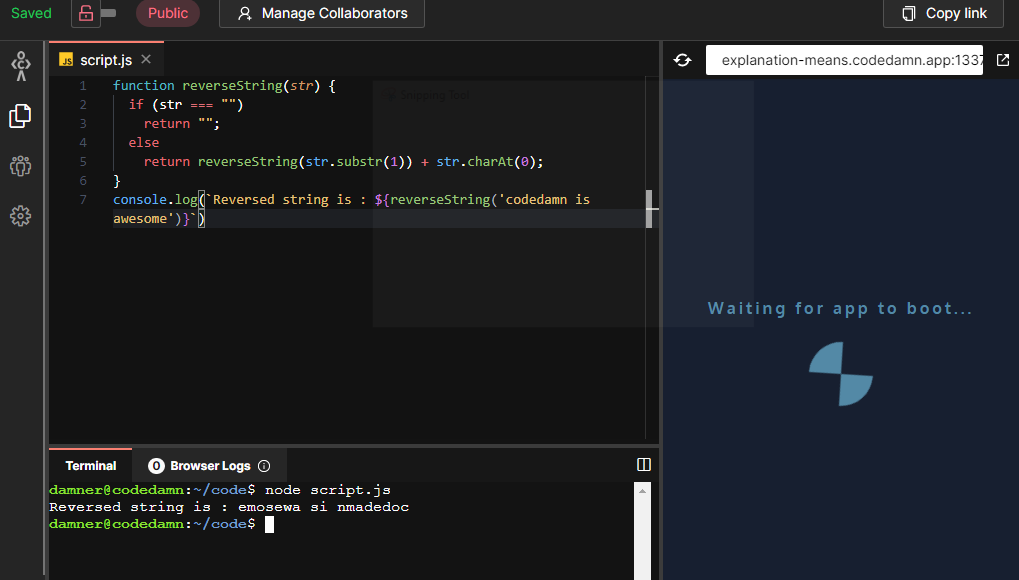
There are various ways to reverse a string in javascript but the common and simple approach to solving this problem is to use the above solutions also you can try to find other ways to achieve the same results.
Conclusion
This was all about how to reverse a string in javascript. Hope you learned something new from this article if you have any queries or suggestions you can write them in the comment section and also do check out the complete javascript course on codedamn.
You can also practice coding on codedamn playgrounds and be a part of the community by joining the official codedamn discord server. All the best!
Sharing is caring
Did you like what Ganesh Patil wrote? Thank them for their work by sharing it on social media.
No comments so far
Curious about this topic? Continue your journey with these coding courses: