Complete guide to inheritance in Java

Java is one of the most popular programming languages in the world and known for its object-oriented programming nature. It has been over two decades since its first release and is now used by a million developers. In this article, we will be learning one of the most important concepts in OOPs known as inheritance in java.
Introduction
Inheritance is an Object Oriented Programming concept in Java where a class takes properties of its parent class. These properties closely resemble our actual world where children take features like hair color, height, etc from their parents. Moreover, it is also known as the IS-A relationship or the parent-child relationship. Let’s look at the following examples to better understand them!
- Windows is an Operating System
- Cat is an animal
- Physics is a subject
- Engineer is an Employee
We can inherit a class using the extends keyword. The new class we build by inheriting the superclass(parent/ base class) is known as the subclass(child/derived class).
class Employee { // parent class
String name;
int salary;
public void printSalary() {
System.out.println("Salary is " + salary);
}
}
// inherit from Employee
class Engineer extends Employee { // child class
public void display() {
System.out.println("My name is " + name);
}
}
class Main {
public static void main(String[] args) {
// create an object of the subclass
Engineer person = new Engineer();
// access field of parent class
person.name = "Rohit";
person.salary = 75000;
// call method of child class
person.display();
// call method of parent class using child class
person.printSalary();
}
}
Code language: Java (java)
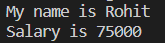
In the above example, the Engineer class inherits properties of the Employee class. We create an object of the child class(Employee) and call methods of that class as well its parent/superclass.
Types
There are three main types of inheritance in Java –
- Single inheritance
- Multilevel inheritance
- Hierarchical inheritance
Single inheritance
A relationship between only two classes such that one class extends from another class is known as single inheritance.
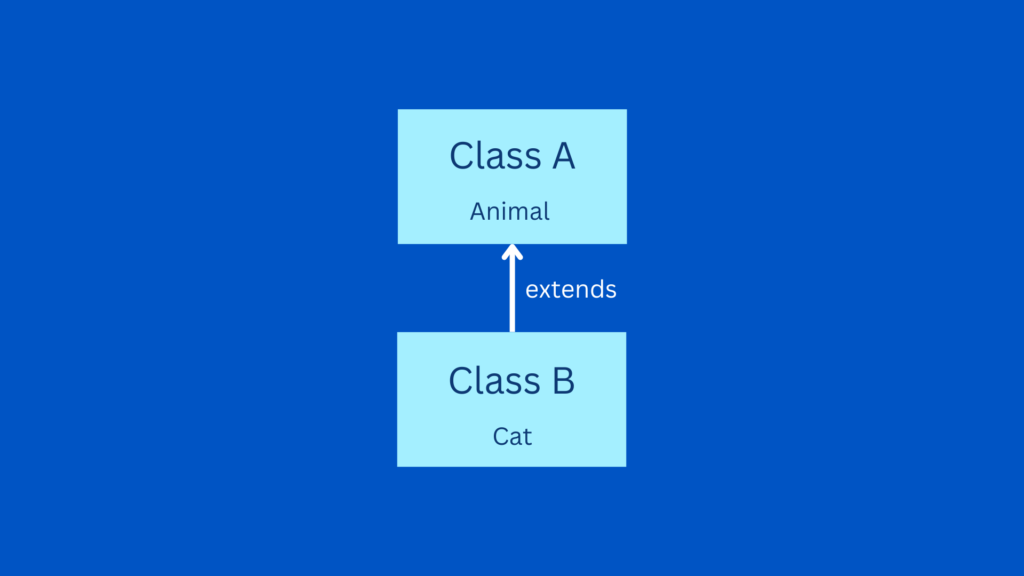
class A { // parent class
public void funA() {
System.out.println('A');
}
}
class B extends A { // child class
public void funB() {
System.out.println('B');
}
}
Code language: Java (java)
Multilevel inheritance
Suppose we have three classes A, B, and C. Multilevel inheritance occurs when a child class C extends from a parent class B, and the parent class B acts as a child class for another parent class A.
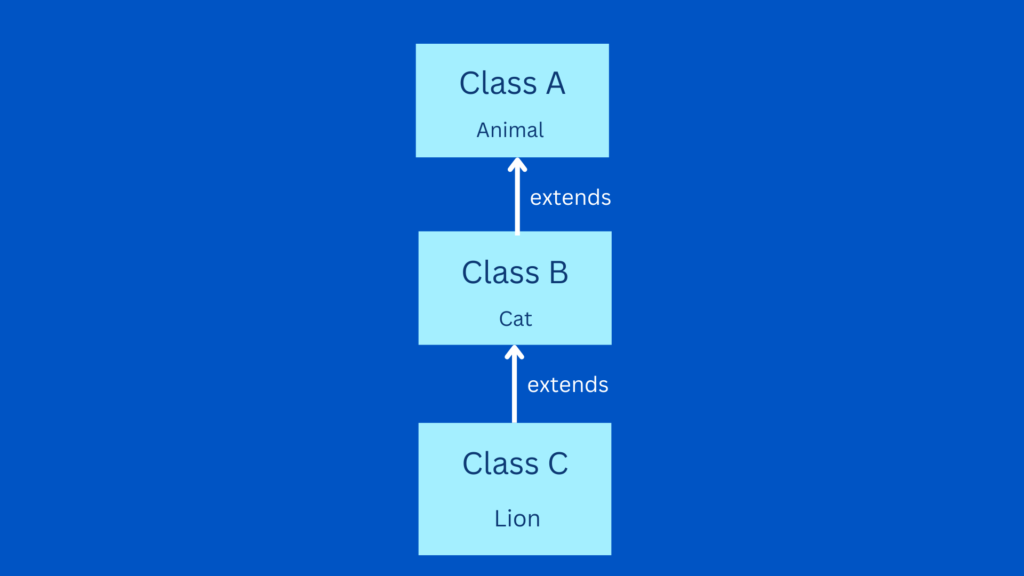
class A { // parent class of B
public void funA() {
System.out.println('A');
}
}
class B extends A { // parent class of C and child class of A
public void funB() {
System.out.println('B');
}
}
class C extends B { // child class of B
public void funC() {
System.out.println('C');
}
}
Code language: Java (java)
The level of inheritance is not limited to three, it can go as much as we want.
Hierarchical inheritance
This type of inheritance occurs when multiple child classes inherit from a single-parent class.
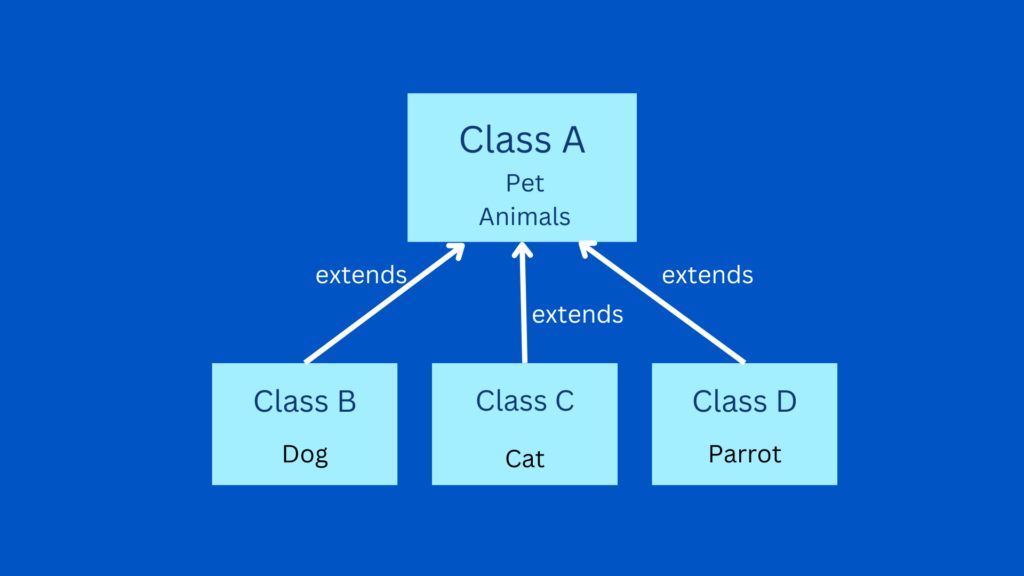
class A { // parent class of B and C
public void funA() {
System.out.println('A');
}
}
class B extends A { // child class of A
public void funB() {
System.out.println('B');
}
}
class C extends A { // child class of A
public void funC() {
System.out.println('C');
}
}
class D extends A { // child class of A
public void funD() {
System.out.println('D');
}
}
Code language: Java (java)
Multiple inheritance
It occurs when a child class inherits from multiple parent classes.
Note: Java doesn’t support multiple inheritance.
Multiple inheritances can cause a lot of runtime errors. For example, if we want to run a method that happens to exist in both the parent classes, then which parent class will Java prefer to run?
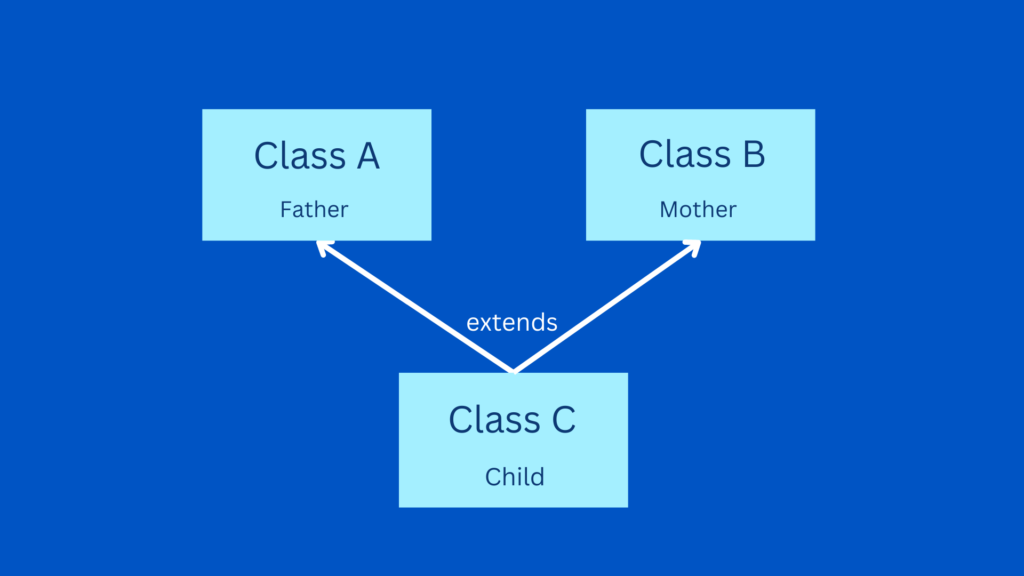
Hybrid inheritance
Hybrid as the name suggests is a combination of 2 or more types of inheritance.
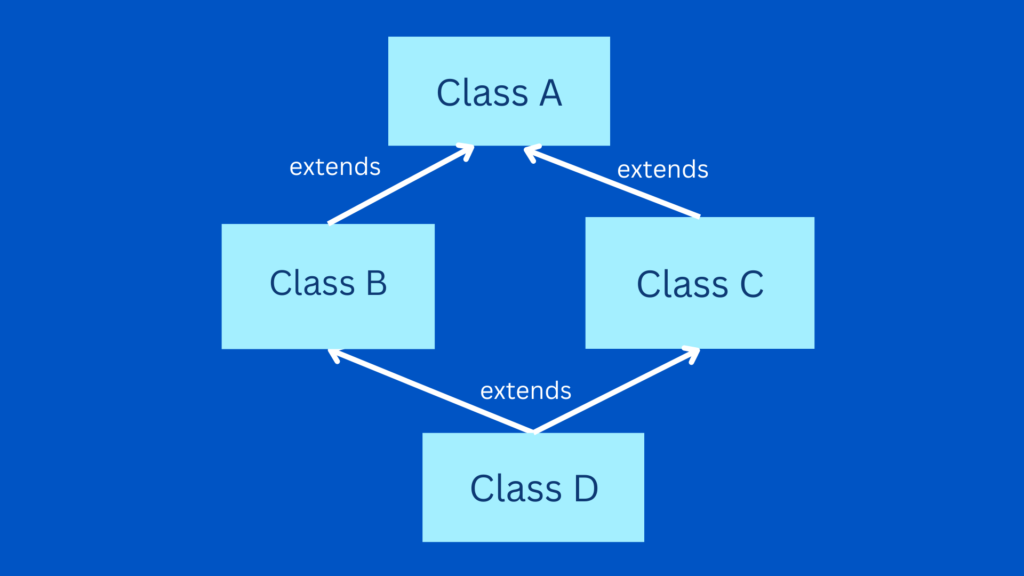
Method Overriding
Sometimes, the parent and child classes have the same method name. Here, we might want to override/change the methods of the parent class so that we can perform the action specified by our child class. Even if we don’t mention @Override
, this is the default behavior.
class A { // parent class
String user = "root";
public void display() {
System.out.println("\nMy name is " + user + '\n');
}
}
class B extends A { // child class
@Override
public void display() {
System.out.println("\nMy name is Rahul\n");
}
}
class Main {
public static void main(String[] args) {
// create an object of the subclass
B obj = new B();
obj.display();
}
}
Code language: Java (java)
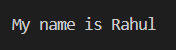
Super keyword
In the above example, we override the parent method. But, what if we want to call the method of the parent class along with the child method? This can be performed by using the super keyword. This act as an object of the parent class which enables us to call methods of the superclass(parent class).
class A { // parent class
String user = "root";
public void display() {
System.out.println("\nMy name is " + user + '\n');
}
}
class B extends A { // child class
public void display() {
super.display();
System.out.println("\nMy name is Rahul\n");
}
}
class Main {
public static void main(String[] args) {
// create an object of the subclass
B obj = new B();
obj.display();
}
}
Code language: Java (java)
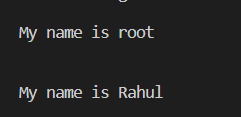
Conclusion
In this article, we learned about various types of inheritance and their various keywords. Apart from that, the inheritance concept is beneficial. It provides features like –
- Method overriding: We can achieve polymorphism in Java using the concept of inheritance.
- Code reusability: Having the ability to use methods/fields of the parent class helps to implement them efficiently.
If you want to learn more about Java, you can start learning Java from a beginner-friendly course on Codedamn here.
Sharing is caring
Did you like what Fidal Mathew wrote? Thank them for their work by sharing it on social media.
No comments so far
Curious about this topic? Continue your journey with these coding courses: