What is SQLite? Everything about SQLite with examples
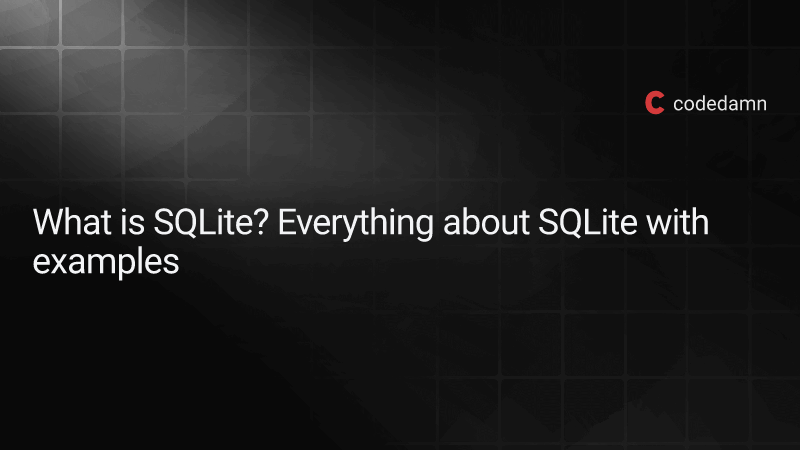
SQLite is a self-contained, high-reliability, embedded, full-featured, public-domain, SQL database engine.
It is one of the most popular databases in the world. Its serverless architecture and zero-configuration requirement simplify development, ensuring SQLite’s wide adoption across a variety of platforms and programming languages.
History of SQLite
SQLite was conceived in the spring of 2000 by D. Richard Hipp, originally designed to meet the database needs of his Fossil SCM. It quickly evolved into a general-purpose library. SQLite’s development has been marked by its adherence to the principles of simplicity, reliability, and independence. Over the years, it has seen substantial growth in features and capabilities, with key milestones including the introduction of the Write-Ahead Logging (WAL) mode for improved concurrency and the continuous enhancement of its query optimizer.
Core Concepts and Features
Zero-configuration
One of SQLite’s hallmark features is its zero-configuration nature. Unlike traditional database systems, SQLite does not require a separate server process or system to operate. This eliminates the need for database installation, setup, and management, making it an excellent choice for developers seeking to build applications with minimal overhead.
Server-less architecture
SQLite’s server-less architecture means that it runs directly within the application it powers. There is no need to communicate with a separate database server, which can reduce latency and complexity. This architecture makes SQLite an integral part of the application, leading to simplified architecture and deployment.
Single-database file and cross-platform support
SQLite stores the entire database (definitions, tables, indices, and the data itself) as a single cross-platform file on a host machine. This singular file approach simplifies data transfer, backups, and portability. SQLite’s cross-platform support extends its utility across various operating systems and environments, making it a versatile tool for developers.
ACID compliance
SQLite ensures full ACID (Atomicity, Consistency, Isolation, Durability) compliance, even in scenarios of system crashes or power failures. This compliance guarantees that all database transactions are processed reliably, maintaining data integrity and consistency.
Embedded and highly portable
Being a self-contained library that requires minimal support from external libraries or operating systems, SQLite is easily embedded into other applications. Its portability is unmatched, with support for most known operating systems and architectures, enhancing its appeal for a wide range of applications.
Language support
SQLite interfaces with numerous programming languages, including but not limited to C, C++, Python, Java, and PHP. This extensive language support, facilitated through various bindings and wrappers, ensures that developers can integrate SQLite into their projects, regardless of the programming environment.
SQLite Data Types
SQLite employs a dynamic typing system, unlike many other SQL databases that require a strict datatype definition. This system, known as “manifest typing,” allows the type of a value to be determined by its content. Primary datatypes in SQLite include INTEGER, TEXT, BLOB, REAL, and NULL, providing flexibility in how data is stored and manipulated.
Basic Operations with SQLite
Setting up SQLite environment
Setting up SQLite involves downloading a precompiled binary from the official SQLite website or compiling it from source. For many environments, it’s as simple as integrating the SQLite library into your project.
Creating a database and tables
To create a database, simply connect to it using a SQLite client or library function. If the database does not exist, SQLite will create it automatically. Table creation follows SQL standards, with statements executed through the SQLite command line or programmatically in your application code.
CREATE TABLE IF NOT EXISTS users (
id INTEGER PRIMARY KEY,
name TEXT NOT NULL,
email TEXT UNIQUE NOT NULL
);
CRUD operations
SQLite supports standard CRUD (Create, Read, Update, Delete) operations through SQL statements. Here’s a quick overview:
- Create: Insert new records into your tables.
INSERT INTO users (name, email) VALUES ('John Doe', 'john@example.com');
- Read: Query data from your tables.
SELECT * FROM users;
- Update: Modify existing records.
UPDATE users SET name = 'Jane Doe' WHERE id = 1;
- Delete: Remove existing records.
DELETE FROM users WHERE id = 1;
SQL transactions
Transactions in SQLite follow the ACID principles, allowing multiple operations to be executed as a single unit of work. Transactions are started with the BEGIN TRANSACTION
command, and can be committed with COMMIT
or rolled back with ROLLBACK
, ensuring data integrity.
BEGIN TRANSACTION;
INSERT INTO users (name, email) VALUES ('Alice', 'alice@example.com');
UPDATE users SET name = 'Alice B.' WHERE email = 'alice@example.com';
COMMIT;
Advanced Features
SQLite boasts a range of advanced features that enhance its performance and utility. Understanding these features allows developers to fully leverage SQLite’s capabilities in their applications.
Indexing
Indexes in SQLite boost query performance by minimizing the number of rows to be scanned to find a particular subset of data. Creating indexes on columns often queried together can significantly enhance the speed of read operations. It’s crucial, however, to use indexing judiciously; while they speed up reads, they can slow down writes and updates due to the additional overhead of maintaining the index.
Triggers
Triggers in SQLite enable automatic execution of specified actions in the database, such as updates or inserts, in response to certain events on a table. They are powerful tools for maintaining data integrity and automating repetitive tasks. For example, a trigger could automatically update a “last_modified” timestamp column whenever a record is updated.
Views
Views in SQLite are virtual tables created by a query. They simplify complex operations by encapsulating sophisticated queries into a single virtual table, making them reusable and easier to manage. Views are not stored as data but are dynamically constructed when accessed, making them a flexible tool for data representation.
Common Table Expressions (CTEs) and Window Functions
SQLite supports Common Table Expressions, providing a more readable and modular approach to writing complex queries. CTEs can be recursive, allowing for elegant solutions to hierarchical data browsing. Window Functions, on the other hand, provide powerful analytical capabilities by performing calculations across a set of rows related to the current row, enabling sophisticated data analysis and reporting within the database.
Working with SQLite in Different Programming Languages
SQLite’s simplicity and broad support make it a popular choice across various programming environments.
Python
Python’s sqlite3
module provides a straightforward interface to SQLite databases. Here’s a basic example of creating a database, inserting data, and querying it with Python:
1import sqlite3
2
3# Connect to database (or create if it doesn't exist)
4conn = sqlite3.connect('example.db')
5c = conn.cursor()
6
7# Create table
8c.execute('''CREATE TABLE stocks
9 (date text, trans text, symbol text, qty real, price real)''')
10
11# Insert a row of data
12c.execute("INSERT INTO stocks VALUES ('2022-01-05','BUY','RHAT',100,35.14)")
13
14# Save (commit) the changes
15conn.commit()
16
17# Query the database
18for row in c.execute('SELECT * FROM stocks ORDER BY price'):
19 print(row)
20
21conn.close()
JavaScript (Node.js)
Node.js applications can interact with SQLite databases using libraries such as sqlite3
. A simple example is shown below:
1const sqlite3 = require('sqlite3').verbose();
2let db = new sqlite3.Database('./example.db', (err) => {
3 if (err) {
4 console.error(err.message);
5 }
6 console.log('Connected to the example.db database.');
7});
8
9db.serialize(() => {
10 db.run("CREATE TABLE IF NOT EXISTS langs (name TEXT)");
11
12 db.run(`INSERT INTO langs(name) VALUES(?)`, ['C'], function(err) {
13 if (err) {
14 return console.log(err.message);
15 }
16 console.log(`A row has been inserted with rowid ${this.lastID}`);
17 });
18
19 db.each(`SELECT * FROM langs`, (err, row) => {
20 if (err) {
21 throw err;
22 }
23 console.log(row.name);
24 });
25});
26
27db.close((err) => {
28 if (err) {
29 console.error(err.message);
30 }
31 console.log('Close the database connection.');
32});
Other Languages Support
SQLite’s wide adoption is partly due to its extensive language support, including but not limited to C/C++, Java, PHP, and Ruby. Each language interface adheres to its conventions while providing access to SQLite’s full feature set, enabling seamless integration into various development environments.
Use Cases and Real-world Applications
SQLite’s versatility finds it in a multitude of applications, from embedded systems to large-scale web applications.
Embedded Systems and IoT Devices
Due to its lightweight nature, SQLite is a perfect fit for embedded systems and IoT devices, where resources are limited. It can manage data locally, providing fast access without the need for a network connection to a centralized database.
Web Browsers and Web Applications
Major web browsers use SQLite for storing client-side data, such as cookies, sessions, and history. Web applications also leverage SQLite for prototyping, testing, and even production environments where the database load is manageable.
Mobile Applications
SQLite is the go-to database for Android and iOS applications, offering an efficient way to store and manage data locally on the device. Its reliability and self-contained architecture make it ideal for mobile app development.
Desktop Applications
Many desktop applications use SQLite for local data storage, from simple utilities to complex software suites. Its portability and ease of use make it a preferred choice for developers.
Analysis of Large Datasets
While not traditionally designed for massive databases, SQLite can be used for analyzing large datasets with the right optimizations. Its simplicity and the ability to run on minimal hardware make it a valuable tool for data analysis in resource-constrained environments.
Performance Optimization and Best Practices
Optimizing SQLite performance involves a combination of best practices and leveraging its features effectively. Some tips include:
- Use transactions to bundle multiple operations, reducing disk I/O overhead.
- Employ indexing strategically to speed up queries while being mindful of write operations.
- Normalize data where appropriate to reduce redundancy and improve query performance, but also consider denormalization in read-heavy scenarios for efficiency.
- Regularly vacuum the database to defragment and optimize storage.
Comparison with Other Database Systems
SQLite’s unique characteristics position it distinctively among other popular databases.
SQLite vs. MySQL
MySQL is a full-featured SQL database server, offering more scalability and concurrency control, suited for large, multi-user applications. SQLite, with its serverless architecture, excels in simplicity, portability, and ease of use for smaller-scale applications or when a lightweight database is needed.
SQLite vs. PostgreSQL
PostgreSQL offers advanced features like full ACID compliance, extensible types, and sophisticated locking mechanisms, making it suitable for complex, high-volume environments. SQLite’s appeal lies in its minimal setup, requiring no dedicated database server, making it ideal for applications where such advanced features are unnecessary.
SQLite vs. NoSQL databases like MongoDB
NoSQL databases like MongoDB provide schema-less data storage, horizontal scaling, and real-time analytics, catering to applications with large volumes of unstructured data. SQLite, being a relational database, is better suited for structured data and applications requiring ACID compliance and SQL capabilities.
Limitations and When Not to Use SQLite
While SQLite is versatile, it’s not always the best choice. Scenarios where SQLite might be less suitable include:
- Applications requiring high write volumes or concurrent writes, as SQLite’s locking mechanism can become a bottleneck.
- Projects needing built-in user management and sophisticated access control.
- Systems where the database size is expected to grow beyond several terabytes, making file-based management cumbersome.
Sharing is caring
Did you like what Mehul Mohan wrote? Thank them for their work by sharing it on social media.
No comments so far
Curious about this topic? Continue your journey with these coding courses: