How to handle React onClick event? Complete Guide with Examples
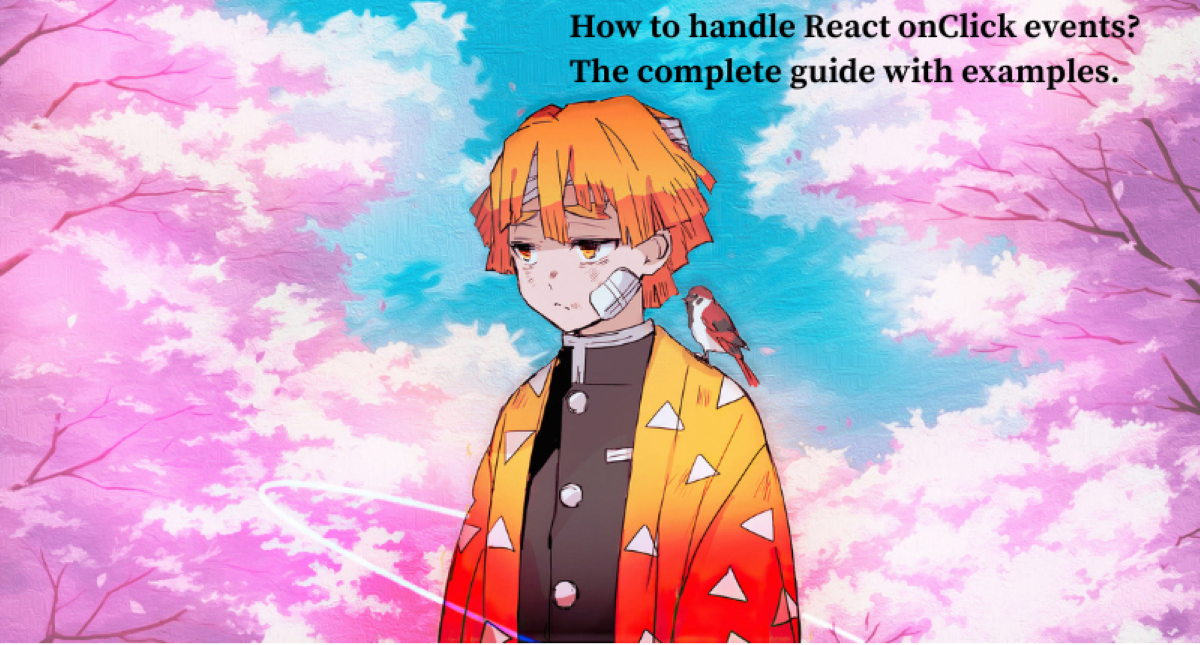
In this article, we will see what react events are, and how to handle react onClick events with examples. Working with events is important when you are creating any project, Like when the user scrolls the page when the user will click a specific button etc. are the basic events which we can add to our projects. So let’s discuss events and how to use them in ReactJs. If you are a newbie in programming or you want to start but don’t know where you can learn to code then you should visit Codedamn.
What are events in React?
When certain changes happen on the webpage or any user interaction and we want to react to that changes we can use events. Events are part of the Document Object Model and it includes so many events for example. If any user clicks on a button we can use a Click event which is present in every button element. Or what we want to show users when they load the webpage in their browsers we can do that with the help of the onLoad event. there are so many events but we will only be looking at the onClick event in this article.
Let’s first see a simple React onChange
event which we can use to handle the user inputs. onChange
event triggers every time the user types anything in the form input. And it stores that value in an event object which we can access in our code using the event
object.

This is how the event object looks it contains all the details about the occurred event. also, the properties which we can use to store the value of the user inputs into our state. Inside the event object, there is a target property inside that property we can see the value of the entered input. It means we are reacting to the user input using the onChange event. Reacting to the user interactions on our webpage and based on their interactions showing them something is what events are.
React onClick events
There are dozens of DOM events present in ReactJS. The onClick event is one of them. We can use the onClick event to handle user clicks and what to show them after that click happen is known as event handling. It is a really important topic which you will need to know as a ReactJS developer. We can handle the clicks event using the onClick
props. the props which start with on
are all known as event props. And they are not user-defined props this is the props which are available on every single JSX element in Reactjs. Which we can use to handle events. As we saw in the above code example image.
Let’s see how can handle onClick events in ReactJS.
How we can handle onClick events in ReactJS?
Handling onClick events are not that hard. If you have worked with Event Listeners in JavaScript then you can work with onClick events in ReactJS easily. Let’s see the JavaScript onclick
event first and then how the onClick event in React works. In JavaScript, we imperatively use events, what does it mean? it means that we have to select the element first then we need to add the event handler on that. After that, we will specify what that event will be doing. It is an imperative way of doing stuff in programming. let’s see this in an example.
const button = document.querySelector('.button');
button.addEventListener('click', () => {
console.log('button clicked');
});
Code language: JavaScript (javascript)
In the above example, I first selected the button and then added the click Event listener to it. Then I passed an anonymous callback function which will execute as soon as the button is clicked. This is how we can handle onclick
events in JavaScript. But now let’s see how we can handle React onClick events.
Handling React onClick events
First of all, for handling the onClick event we will be needing a button element. so let’s create a button component and add a button element in there. At first, we will not be going to click the button but then we will add the onClick event to the button and increment the number of times the button clicked happen on the page. As shown in the code example image below.

When working with react events keep in mind that we don’t use the onclick
in small case but we use it as a camel case that’s the thing you need to keep in mind. Let’s get back to our example. In the above example image, we created a button element in our App.js component and added an onClick property to it. remember it is not the prop that we added on our own. It’s a DOM property that is available on every JSX element.
After that, I passed the clickHandler function to the onClick event. I don’t invoke the function there I just passed the function. This means when I will click on that button the code will execute and it will check if there are any properties present on the button element. If yes then that clickHandler function will be going to execute. Let’s now see this onClick handler in our console.
onClick events into our console

As you can see I have clicked the button element 5 times and I was able to count how many times the click happened. Because of the state which was changing each time the click was happening and you can see the message on the console also. And this happens only because we were able to react to that click event with the help of the onClick event. There are so many use cases of the events in the modern web world. You should learn them before even starting ReactJS.
Example
Let’s see some examples of the onClick events in ReactJS. In the first example what I want to do is. I want a button which can change the background colour of the UI with every click just some random colour. Let’s do that in practice.
For doing that we will first need a button element. Into our component. After adding that button element we will then create a colours state which will hold an array of different colours. And what I want now is I want to create a random colour every time the user click happens. As shown in the code example below.
import { useState } from "react";
import "./styles.css";
const randomColors = [
"#FF6633",
"#FFB399",
"#FF33FF",
"#FFFF99",
"#00B3E6",
"#E6B333"
];
export default function App() {
const [color, setRandomColor] = useState(randomColors[0]);
const clickHandler = () => {
const randomColor = Math.ceil(Math.random() * randomColors.length);
setRandomColor(randomColors[randomColor]);
};
return (
<div className="App" style={{ backgroundColor: color }}>
<button onClick={clickHandler}>random</button>
</div>
);
}
Code language: JavaScript (javascript)
Here, in the above code example, I first created a button element and on that button, I added the onClick event and as a callback function, I passed the clickHandler function to it. Now every time the click happens on that button the onClick event will be triggered and as soon as the onClick event will be triggered the callback function will execute. and the code inside that clickHandler function will also be going to execute. And as I set the color
state to the random
colors
. On every click, the setColor setter will set a random colour every time the clickHandler function will execute. This is a small but really helpful example for understanding the onClick event in ReactJS.
Different ways to handle onClick event
Now we saw how to handle the onClick event in ReactJS with examples. Let’s now see some different ways to handle the onClick event. so the first one is passing a click handler function as a reference.
Passing a click handler function
The most used way is to create a click handler function and pass it to the onClick event. As soon as the event will trigger a button the click handler function will be going to execute. And what we have written inside that click handler function will also be going to execute. I prefer using this way every time I work with events. You can use something that you like also. As shown in the example below.
export default function App() {
const [numClick, setNumClick] = useState(0);
const clickHandler = () => {
setNumClick(numClick + 1);
};
return (
<div className="App">
<p>Passing click Handler example</p>
<h1>{numClick}</h1>
<button onClick={clickHandler}>Click me</button>
</div>
);
}
Code language: JavaScript (javascript)
Passing an anonymous callback function
The second way to handle the onClick event is to pass an anonymous callback function directly inside the onClick prop. Then whenever the click will happen on the button the anonymous callback function will be going execute. This is also an easy way without even creating any extra code block for the function we can handle the onClick event. As shown in the example below.
export default function App() {
const [numClick, setNumClick] = useState(0);
return (
<div className="App">
<p>Passing anonymous function example/p>
<h1>{numClick}</h1>
<button
onClick={() => alert("Click happened")}>Click me
</button>
</div>
);
}
Code language: JavaScript (javascript)
Updating state directly inside the onClick event
now what we can do is we can directly update the state inside the onClick event. consider the click handler example in that example I have created a click handler function and that clickHandler function not doing too much. It’s just updating the click count every time the onClick event triggering.
So we can do that even without adding any extra clickHandler function we will just update the state here inside the onClick event using that state setter function which Reacts useState
hook returns.
We will call that setter function inside the onClick event and that setter function will invoke every time the button click will happen.
As shown in the example below.
import { useState } from "react";
import "./styles.css";
export default function App() {
const [numClick, setNumClick] = useState(0);
return (
<div className="App">
<p>Passing click Handler example</p>
<h1>{numClick}</h1>
<button
onClick={() => {
setNumClick(numClick + 1);
}}
>
Click me
</button>
</div>
);
}
Code language: JavaScript (javascript)
Conclusion
That’s it about our article, How to handle React onClick events? In this article, we talked about what react events are, and how to handle them and we also see them in practice. I hope you find this article useful if you want to start your career as a programmer or you started programming but don’t know from where you can learn. Then you should visit Codedamn there you can learn and practice both at the same time.
Sharing is caring
Did you like what Amol Shelke wrote? Thank them for their work by sharing it on social media.
No comments so far
Curious about this topic? Continue your journey with these coding courses: