What are Tuples in Python? Complete guide with examples
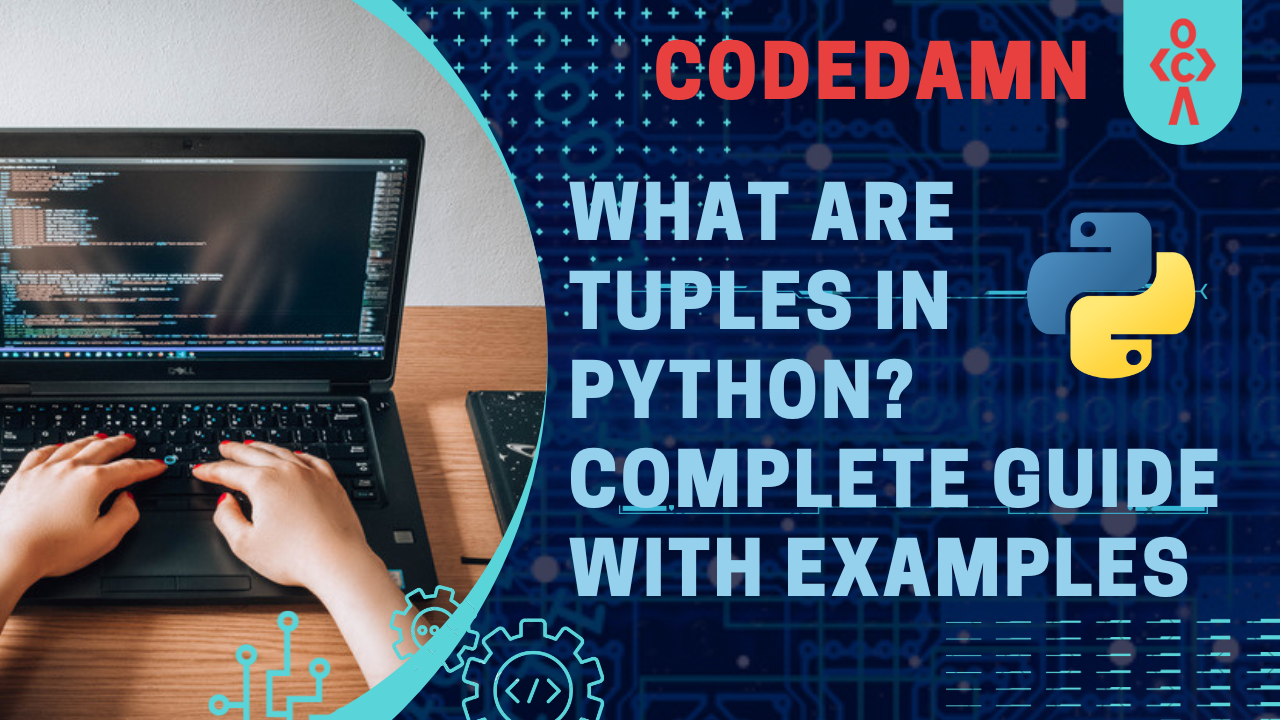
You must be familiar with Lists in Python, Just like it, there is another sequence data type in Python known as Tuples. They look similar but have a great difference in their structure and working. Wanna know them? Let’s get started with it.
Introduction
A tuple is a data structure in Python that looks like a list but differs in the fact that you cannot modify its contents once you create them. A tuple is a comma-separated sequence of data enclosed in round brackets. In this article, we will be discussing all the knowledge you would need to understand tuples in Python, from creating them to accessing and manipulating its elements, at the end, we will also cover some advantages and disadvantages of using tuples.
Some key elements of a tuple include:
- Just like in lists, in a tuple, you can insert different data types, from integers to boolean values to strings, and even other tuples.
- A tuple can have a variable length, ranging from zero elements to as many as desired.
- You can add a tuple inside a tuple, and this is called the nesting of tuples.
Creating a Tuple
The most common way among the several different ways of creating a tuple is to enclose a comma-separated list of elements in parentheses. For example:
tuple_int = (22,98,54,632)
tuple_str = ('Tushar', 'BuntY', 'raKESh')
tuple_bool = (True, False, True, False, True)
print(tuple_int,'\n',tuple_str,'\n',tuple_bool)
Code language: Python (python)

You can also use the built-in function tuple()
. This will create a tuple from sequences of data, such as a list or a string. For example:
tup_1 = tuple([22,98,54,632])
tup_2 = tuple('a0b0c')
tup_3 = tuple((True, False, True))
print(tup_1,'\n',tup_2,'\n',tup_3)
Code language: Python (python)

You can also create a tuple with just one element. If you don’t believe it, then try to create a tuple with a single element and just include a comma after the first element. This is necessary because, in Python, we use parentheses to indicate a tuple even if it only has one element. For example:
one_tup_1 = (99,)
one_tup_2 = ('Ram',)
one_tup_3 = (True,)
print(one_tup_1,'\n',one_tup_2,'\n',one_tup_3)
Code language: Python (python)

Accessing Tuple Elements
After creating the tuple, you must want to display the elements from it. You can access or traverse through these elements using indexing or slicing.
Using Indexing
One of the most popular functions in python that is used to display a particular element from an array, list, tuples, dictionary, etc is none other than our famous, indexing function, To perform indexing, we use the square brackets []
and mention the index of the element you want to access. The index of the first element in Python starts at 0, with a constant increase in the index of 1 (i.e., the index of the second element is 1), and so on. For example:
tuple_print = (34, 'You', int(True), 98)
print(tuple_print[0]) #this statement prints 34
print(tuple_print[1]) #this statement prints You
print(tuple_print[2]) #this statement prints 1
print(tuple_print[3]) #this statement prints 98
Code language: Python (python)

Just as we can access elements from the left side to the right side of the tuple, You can also use negative indices to access elements from the right side to the left side i.e. from the end of the tuple. For example:
tup_1 = (34, 'You', int(True), 98)
print(tup_1[-1]) #This statement prints 98
print(tup_1[-2]) #This statement prints 1
print(tup_1[-3]) #This statement prints You
Code language: Python (python)

Using Slicing
Python provides a function that we can use to traverse along a range of elements, this is possible with the help of slicing. To perform slicing we can use the colon :
operator and then specify the indices on the left and right to the colon in the order of the first and last elements you want to include in the slice. For example:
tup_slice = (97, True, 11, 4)
print(tup_slice[0:2]) # prints (97, True)
print(tup_slice[1:3]) # prints (True, 11)
print(tup_slice[1:]) # prints (True, 11, 4)
Code language: Python (python)

Using for Loop
You can also use the for loop to traverse through the elements of a tuple. For example:
loop_tup = (100, 99, 98, 97)
for i in loop_tup:
print(i)
Code language: Python (python)

Some Features of Tuples
Python offers some in-built functions which you can use to carry out operations like finding the type of object, length of the object, etc. Let’s now discuss a few.
Length of Tuple
We use the len()
function to print the length of a tuple, which is the number of elements it contains. For example:
len_of_tup = (50, 500, 5000, 50000)
print("Length of the Tuple is: ",len(len_of_tup)) #This will print 4
Code language: Python (python)

Class of Tuple object
The type()
function can be used to determine the class of a tuple object. For example:
class_tuple = (100, 101, 1, 2)
print(type(class_tuple))
#This will print <class 'tuple'>
Code language: Python (python)

Operations on Tuples
We have talked about what a tuple is, how to create it, and traverse its elements, along with some extra features. It’s time we start with manipulating the elements present inside a tuple. But we know that tuples are immutable, so it might not be possible to modify (update values, delete values, add new values) their elements directly. However, there is a loophole that makes these operations possible. It is achieved by creating a new tuple with the desired elements.
Updating Tuples
To update a tuple, you can use the concatenation operator + to create a new tuple that combines the elements of the original tuple with the elements you want to add or modify. For example:
tup_1 = (1, 3, 9, 11)
tup_2 = tup_1[:2] + ('Five', 'Seven') + tup_1[2:]
print(tup_2) #This will print (1, 3, 'Five', 'Seven', 9, 11)
Code language: Python (python)

Joining Tuples
If you want to concatenate/join two or more different tuples, you can use the ‘+’ operator. This operator joins the two same data type structures and which in turn creates a new tuple that combines the elements of the original tuples. For example:
add_tup_1 = ('One', 2, 'Three')
add_tup_2 = (4, 'Five', 6)
joint_tup = add_tup_1 + add_tup_2
print(joint_tup)
#This prints ('One', 2, 'Three', 4, 'Five', 6)
Code language: Python (python)

Deleting Tuples
The next function on our tuple is the function to remove or delete a tuple. You can use the del
statement for the same, it completely deletes the tuple including its structure and data inside it. This also removes the tuple from memory and frees up the memory space it was occupying. For example:
tup_del = (2,46,21,22)
del tup_del
print(tup_del)
#this will print error if the tuple is deleted
Code language: Python (python)

Slicing Tuples
To slice a tuple, you can use the colon :
and specify the indices of the first and last elements you want to include in the slice and store them in a new variable. For example:
tup_slice = (97, True, 11, 4, 'qwerty', 'food')
slice_1 = tup_slice[0:2]
slice_2 = tup_slice[3:]
print(slice_1)
print(slice_2)
Code language: Python (python)

Advantages and Disadvantages of Using Tuples
Now that we are familiar with tuples let’s talk about the advantages and disadvantages this data structure has to offer.
Advantages of Tuples:
- Tuples are more memory-efficient than lists since they do not require extra space for storing the list’s size and capacity.
- Tuples are faster than lists when it comes to accessing and iterating over their elements because they take less memory than lists.
- Tuples provide an additional level of security since they cannot be modified once created. This can be useful in situations where you want to ensure that the data is not accidentally or maliciously changed.
Disadvantages of Tuples:
- Since tuples are immutable, this makes it more difficult to add or remove elements from them.
- Tuples do not have as many built-in methods and functions as lists, which can make them less convenient to work with in some cases.
Conclusion
It’s time we conclude our article today. We discussed tuples in Python, which is a data structure that is similar to lists but is more concise and immutable. You can create tuples using parentheses or the tuple() function, and they can contain elements of different data types. You can access tuples using indexing and slicing and use built-in functions and methods to determine their length and class. While tuples are not as flexible as lists, they provide an additional level of security and can be useful in situations where you want to ensure that the data is not accidentally or maliciously changed.
I hope you found my article helpful; if you have any queries, you can comment down below, and we’ll be back with another informative article. Until then, keep coding, and have a wonderful day ahead!
Sharing is caring
Did you like what Pravin Gupta wrote? Thank them for their work by sharing it on social media.
No comments so far
Curious about this topic? Continue your journey with these coding courses:
308 students learning
Haris
Python Crash Course for Beginners
Shubham Sarda
Python For Absolute Beginners - Learn To Code In Python