Conditionals in PHP (if, else, if-elseif)

PHP, an acronym for Hypertext Preprocessor is one of the most widely used scripting languages. It is easy-to-learn, free, and open-source licensed software. A PHP file has the extension “.php” and more than 70% of the internet comprises PHP-powered backend websites. In this article, we are going to learn about if
, else
, and if-elseif
conditional statements in PHP. If else is one of the most basic programming concepts that are very crucial to learn when getting started with programming.
What are conditional statements?
In day-to-day life, we have to make decisions about what we have to do. For example, do I watch a movie or should I study for my exams? We always ask ourselves questions when faced with a decision before deciding the best course of action in the situation. We use if-else statements in programming languages to accomplish such scenarios.
The decisions are made based on the outcome of a condition whether it is true or false. We use the following logical conditions in PHP to build conditions for our program to check:
- Equal to:
a == b
- Not Equal to:
a != b
- Less than:
a < b
- Less than or equal to:
a <= b
- Greater than:
a > b
- Greater than or equal to:
a >= b
Now, let’s take a look at how if statements work by using these conditions!
If
An if-else statement is a conditional statement that decides the execution path based on whether a specific condition is true or false.
if ( condition )
{
statement; // For true case.
}
Code language: C++ (cpp)
If the condition turns out to be true, we perform the if
statement otherwise no action is performed. Now, let’s take a look at an example.
<?php
$n = 20;
if ($n % 2 == 0)
print($n . " is divisible by 2");
?>
Code language: PHP (php)

In the above example, we are checking whether the number is divisible by 2 or not. First, we declare a variable $n
equal to 20. Then, we check $n%2==0
, i.e whether the number is divisible by 2 or not.
Suppose, I want to check whether the number is odd or even. We would use the same logic here, but the problem is we can only find whether the number is even or not using if
statements. Here’s where we would use the else
statements.
Else
An else
statement as the name suggests only occurs when the condition is not satisfied. The else
clause is optional, i.e it’s not necessary, we can omit the else clause depending on our needs.
if ( condition )
{
statement1; // For true case.
}
else
{
statement2; // For false case.
}
Code language: C++ (cpp)
Now, let’s use the if
and else
statements to check whether the number is even or odd.
<?php
$n = 20;
if ($n % 2 == 0)
print($n . " is even");
else
print($n . " is odd");
?>
Code language: PHP (php)
Test inputs:
$n = 20
Code language: PHP (php)
$n = 45
Code language: PHP (php)
Elseif
The elseif
statement is a combination of both if
and else
statements. The elseif
statement also includes a condition like an if
statement. And, this statement only occurs if the preceding if
statement is false, and the elseif
condition is true.
if ( condition1 )
{
statement1; // If condition1 is true
}
else if (condition2)
{
statement2; // If condition1 is false
// and condition2 is true
}
else
{
statement3; // If both conditions are false
}
Code language: C++ (cpp)
Now, let’s take an example of the student’s marks. In this scenario, if the student gets more than or equal to 90, the student gets a medal. And, if the student gets marks between 89 to 40, the student is declared as passed and moves on to the next class. And finally, if it’s none of the other two cases, it means the student got less than 40. In this case, the student is declared as failed and needs to repeat this class.
<?php
$marks = 80;
if ($marks >= 90)
print("Student gets a medal!");
else if ($marks < 90 && $marks >= 40)
print("Student passed!");
else
print("Student failed!");
?>
Code language: PHP (php)
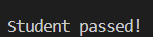
The student passed because the mark of the student is 80 which lies in the region of less than 90 and more equal to 40.
Conclusion
In conclusion, we can say if-else statements are used for decision-making. They are one of the core programming concepts that you learn when you start programming. We use if, else, and else if statements according to our needs as we saw in the above-mentioned examples. You can check out more articles similar to this on the Codedamn platform. Thank you for reading 🙂
Sharing is caring
Did you like what Fidal Mathew wrote? Thank them for their work by sharing it on social media.
No comments so far
Curious about this topic? Continue your journey with these coding courses: