Fix ReferenceError: document is not defined in JavaScript
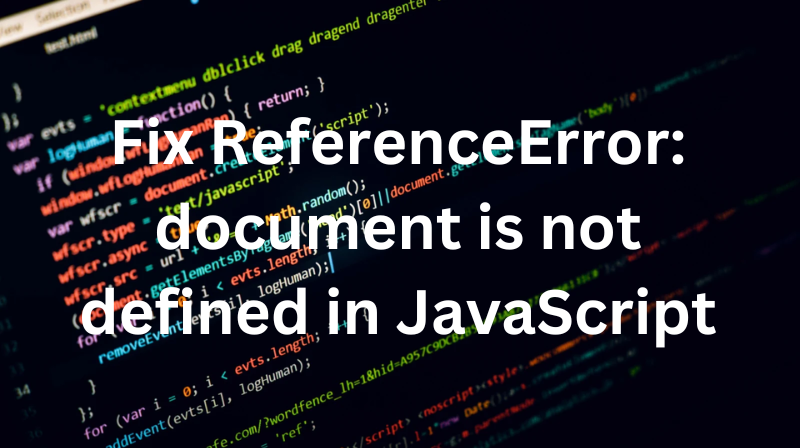
JavaScript forms an inseparable part of nearly every web development application. It adds dynamic to the plain HTML + CSS webpage and allows users to engage. But developers often face many errors because of JavaScript’s involvement with the client and the server. In this article, we will go over the uses of JavaScript in web development, a bit of client-server model introduction, and what exactly is client-side and server-side and the error: “ReferenceError: document is not defined” and see solutions to fix it.
Use of JavaScript
Take a look at the image below:
Are we getting a slightly different vibe than the usual Chrome search page? Yes! But why is that so? The search bar looks different, and the results look a bit funny. We have captured this image after disabling JavaScript in Chrome. The request we sent (we will cover this later) returned a complete webpage. The webpage only displays HTML and CSS after omitting JavaScript.
Whoa! A completely different story here! JavaScript completely changes the outlook of a page. It improves its look and feels and participates in user engagement. Users click on a website link, click on different tabs, hover over tabs and images, and so on. Thus, nearly all web development stacks (like the MERN stack) bundle HTML, CSS, and JavaScript.
Client-side and server-side
We use the terms client-side and server-side to refer to the place where the web application will execute. To give a brief glossary of the client-server model, we classify the devices on the network into clients and servers. The end-user applications, such as mobiles, desktops, and laptops, become the clients. And the large systems handling multiple clients at the same time comprise the servers. Like in the real world, the client “requests” a piece of information by sending an API call to the server. The server, in turn, sends a “response” with the appropriate payload back to the client.
Client-side implies that the web application executes on the client’s browser. All the text, images, user actions, and all the application’s activities run on the client side. On the other hand, server-side means everything mentioned above occurs on the server side.
Reasons for the error
Let’s take a look at the error:
ReferenceError: document is not defined
Code language: Bash (bash)
Using Node.js
Node.js is a server-side runtime. It is mainly in charge of handling the backend of the web application. Therefore, Node.js can do little with the actual DOM manipulation of the webpage on the client side. Thus, we encounter this error when accessing “document” on Node. Node.js does not access the document object, so referencing it causes this error. We do not have a particular workaround for this issue as we are trying to use a “front-end” code on a “backend” situation, which is improper practice. I suggest inspecting your code and figuring out why you need the “document.”
This cause, although discussed for Node.js, applies to all server-side architectures like ASP.NET, PHP, and so on. While using any of them, we can come across this error. So, the best way to prevent this is to ensure we are not executing any front-end code on a backend framework.
Browser issue
You will say that we are executing the front-end code in the browser itself. Then why should we face this error? This situation may arise if we access the document object too early. When the browser loads the webpage, it first creates a base HTML where we mount the JavaScript. Now at this point, we load up the entire web application along with the Document Object Model (DOM) as well. So we will face this error when accessing the document object before this web application is mounted.
As we know, the document is an in-memory representation of the DOM. Therefore it will exist if and only if the DOM itself exists. The most common reason this happens in general code is if we define the <script>
tag before the <body>
ends in the HTML file. I recommend defining the <script>
tag in the body just before it ends. This will ensure that the HTML loads before mounting the <script>
. Therefore, the DOM is ready on the browser end for the JavaScript to manipulate.
<body>
<!-- General HTML code -->
<script type="module" src="index.js"></script>
</body>
Code language: HTML, XML (xml)
Catching the error
Here we will discuss ways to catch this error so that you know exactly when and where to look for it. If we execute the code on the front end, and the DOM exists, so will the document object exist. Therefore, in other scenarios, if we look closely at the error, it says: document is not defined. Thus, the document object value will be “undefined.” We can check this by console logging the value of the document (always a tremendous debugging practice!) We utilize this to check for this error and catch it.
Using just a simple if-else statement, we will be able to check for this error. We can do this in the code modeled below:
if (document !== undefined) {
// your desired code. This is equivalent to a general "try" block
}
else {
// the error code you need to execute when document is undefined. This is equivalent to a general "catch" block
}
Code language: JavaScript (javascript)
We will add our code to execute inside the if block. This is so because the document object value comes out to be undefined. Therefore, we can be sure that the error “document is not defined” will not be raised. On the other hand, if the value does come out to be “undefined” for some reason, then we will execute the else block. We use our error code if we need something precisely executed in such a scenario. Otherwise, we can raise an error to alert the user about the issue. I highly recommend you use this structure for error debugging, not only in this specific case but also in a generic setting.
Conclusion
To conclude, I suggest taking preventive measures to be alert to these errors. It can be a real headache to start debugging the error source in an extensive application (personal experience XD). Please do not use any front-end code on your backend system, as it will undoubtedly throw an error. Only declare your <script> tag after the document is ready. Try understanding the client-server model’s basics to appreciate this error’s necessity fully. Also, always use a try-catch or if-else block to catch these errors. Research more about any errors you face and always Google your queries because the internet has an enormous resource for every issue you face. Happy Coding!
Sharing is caring
Did you like what Sanchet Sandesh Nagarnaik wrote? Thank them for their work by sharing it on social media.
No comments so far
Curious about this topic? Continue your journey with these coding courses: