An In-Depth Guide to Transferring Files from Client to Server Socket in Java
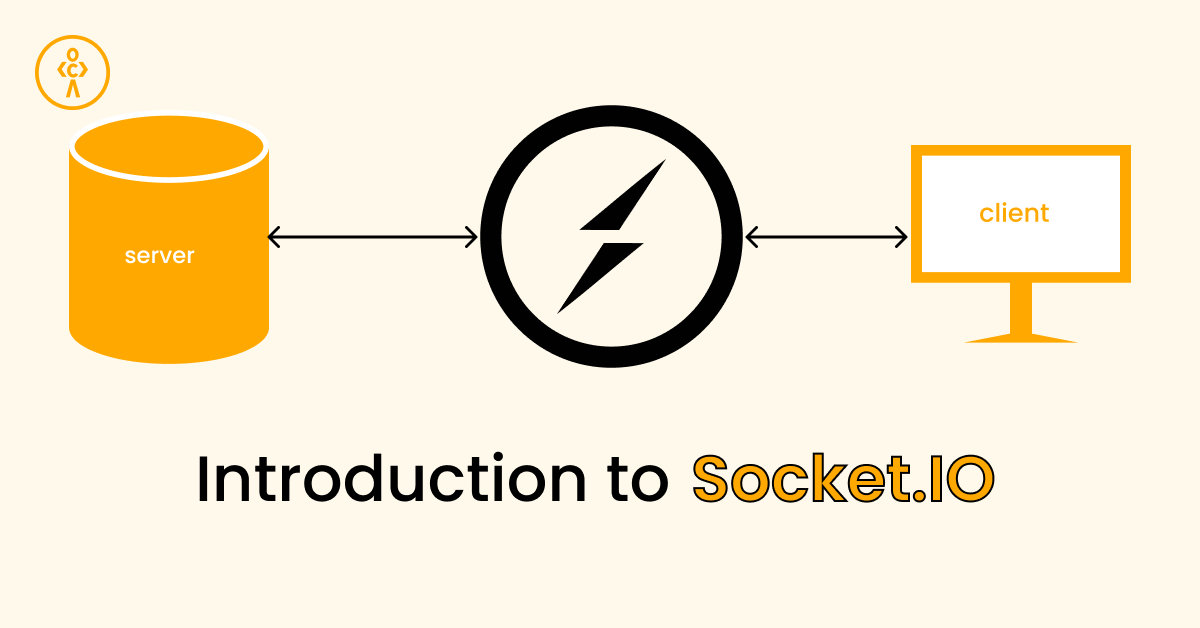
As a programmer, you must be familiar with different methods of transferring data from one system to another. Socket programming in Java is one of the most popular methods which is used to transfer data from one system to another. Socket programming is a type of network communication that allows two systems to communicate with each other over a network. In this guide, we will explore how to transfer files from a client to a server socket in Java. We will cover the basics of socket programming in Java, including how to handle files and program sockets in Java. By the end of this tutorial, you will have a better understanding of how to transfer files between systems using socket programming in Java.
Socket Programming in Java
Socket programming allows two systems to communicate with each other using a network connection. This type of programming is used to transfer data from one system to another. In order to use socket programming in Java, one system should be the client and the other should be the server. The client is responsible for sending out the request to the server, while the server is responsible for responding to the request. In socket programming, the client initiates the communication by establishing a connection with the server and sending out a request. The server then receives the request and processes it, before sending a response back to the client. This back-and-forth exchange of requests and responses forms the basis of socket communication. In order to establish a connection between the client and the server, both systems must be running the same application. Once the connection is established, both systems can then exchange data.
Socket programming in Java is an essential part of network programming, as it enables two systems to communicate with each other over a network. This type of communication is used in many real-time applications, such as online chat, multiplayer games, and file transfer.
File Handling in Java
In order to transfer files from one system to another, we need to use file handling in Java. File handling is a crucial part of any programming language, as it allows applications to read and write data to and from files. In Java, file handling is performed using the java.io package, which provides a set of classes and methods for reading, writing, and manipulating files. Files can be of different types, such as text files, binary files, and so on.
In order to read and write data from a file in Java, we need to use the FileInputStream
FileOutputStream
classes from the java.io package. These classes provide the basic functionality for reading and writing data from and to files in Java. The FileInputStream
class is used to read data from a file, while the FileOutputStream
class is used to write data to a file. Both of these classes provide methods for performing the basic operations of file handling in Java, such as opening and closing files, reading and writing data, and seeking to specific positions in the file We can also use the BufferedReader and BufferedWriter classes to read and write data from a file. These classes provide a more efficient way of reading and writing data to a file.
How to Program Socket in Java
Now that we have discussed socket programming and file handling in Java, let us now look at how to program sockets in Java. In order to program sockets in Java, we need to use the Socket class from the java.net package. The Socket class is used to create a socket and establish a connection between the client and the server. Once a connection has been established, we can use the OutputStream and InputStream classes to send and receive data between the client and the server. The OutputStream class is used to write data to the socket, while the InputStream class is used to read data from the socket.
Programming on the client side
Now that we have discussed how to program sockets in Java, let us now take a look at how many clients can connect to a server socket. The number of clients that can connect to a server socket depends on the type of server socket that is being used. For example, if the server socket is an HTTP server socket, it can handle a maximum of five concurrent connections. On the other hand, if the server socket is a TCP server socket, it can handle up to 256 concurrent connections.
Establish a Socket Connection
To establish a socket connection in Java, you need to import the java.net package and use the Socket class. First, you need to specify the hostname and port number of the server you want to connect to. Then, you can create a new socket object and pass in the hostname and port as arguments. This will establish a connection to the server. You can then use the getInputStream()
and getOutputStream()
methods to send and receive data through the socket connection. It is important to remember to close the socket connection when you are finished using it to free up system resources.
Communication
Communication between connections can occur through a socket connection in Java using the getInputStream
() and getOutputStream()
methods. The getInputStream()
the method allows you to read data that has been sent from the server, and the getOutputStream()
method allows you to send data to the server. Once you have established a socket connection and have access to these streams, you can use the read()
and write()
methods to communicate with the server. It is important to remember to close the connection when you are finished using it to free up system resources.
Closing the connection
To close the connection in Java, you can use the close() method of the Socket class. This will close the connection and release any system resources that were being used by the connection. It is important to always close the connection when you are finished using it, as leaving it open can lead to resource leaks and other problems. It is also a good practice to wrap the close()
the method in a try-catch block in case there are any exceptions thrown when attempting to close the connection.
Programming for the server
Programming for the server in Java involves creating a server socket and binding it to a specific port number. The server socket listens for incoming client connections and creates a new socket for each one. The server can then communicate with the client through the input and output streams of the socket. It is important to properly manage the client connections and make sure to close them when they are no longer needed to free up system resources. The server can also implement multi-threading to allow for multiple client connections to be handled concurrently.
Set up a socket connection
To set up a socket connection in Java, you will need to import the java.net package and use the Socket class. First, you need to specify the hostname and port number of the server you want to connect to. Then, you can create a new Socket object and pass in the hostname and port as arguments. This will establish a connection to the server. You can then use the getInputStream()
and getOutputStream()
methods to send and receive data through the socket connection. It is important to remember to close the socket connection when you are finished using it to free up system resources. Here is an example of how to set up a socket connection in Java:
import java.io.*;
import java.net.Socket;
public class Client {
private static DataOutputStream dataOutputStream = null;
private static DataInputStream dataInputStream = null;
public static void main(String[] args)
{
// Create Client Socket connect to port 900
try (Socket socket = new Socket("localhost", 900)) {
dataInputStream = new DataInputStream(
socket.getInputStream());
dataOutputStream = new DataOutputStream(
socket.getOutputStream());
System.out.println(
"Sending the File to the Server");
// Call SendFile Method
sendFile(
"/home/dachman/Desktop/Program/gfg/JAVA_Program/File Transfer/txt.pdf");
dataInputStream.close();
dataInputStream.close();
}
catch (Exception e) {
e.printStackTrace();
}
}
// sendFile function define here
private static void sendFile(String path)
throws Exception
{
int bytes = 0;
// Open the File where he located in your pc
File file = new File(path);
FileInputStream fileInputStream
= new FileInputStream(file);
// Here we send the File to Server
dataOutputStream.writeLong(file.length());
// Here we break file into chunks
byte[] buffer = new byte[4 * 1024];
while ((bytes = fileInputStream.read(buffer))
!= -1) {
// Send the file to Server Socket
dataOutputStream.write(buffer, 0, bytes);
dataOutputStream.flush();
}
// close the file here
fileInputStream.close();
}
}
Code language: Java (java)
Click here to try this code by yourself.
Communication process
The communication process between a client and a server using a socket connection in Java involves the following steps:
- The client establishes a connection to the server by creating a new Socket object and specifying the server’s hostname and port number.
- The client and server exchange data through the input and output streams of the socket connection. The client can use the
getOutputStream()
method to send data to the server, and the server can use thegetInputStream()
method to receive data from the client. - The client and server can continue to send and receive data as needed.
- When the communication is finished, the client closes the socket connection by calling the
close()
method of the Socket class. This releases any system resources that were being used by the connection.
It is important to properly manage the socket connection and make sure to close it when it is no longer needed to avoid resource leaks and other problems.
Close the Connection
To close the connection in Java, you can use the close()
method of the Socket class. This will close the connection and release any system resources that were being used by the connection. It is important to always close the connection when you are finished using it, as leaving it open can lead to resource leaks and other problems. It is also good practice to wrap the close()
method in a try-catch block in case there are any exceptions thrown when attempting to close the connection. Here is an example of how to close the connection in Java:
<code>// Import the java.net package
import java.net.*;
// Create a Socket object and establish a connection to the server
Socket socket = new Socket(hostname, port);
try {
// Send and receive data through the socket connection
// ...
// Close the socket connection when you are finished using it
socket.close();
} catch (IOException e) {
// Handle any exceptions thrown while closing the connection
// ...
}</code>
Code language: Java (java)
Click here to try this code yourself
File Transfer Implementation in Java Socket
Let us now look at how to implement file transfer using Java socket programming. The below code example demonstrates how to send a file from the client to the server using Java Socket Programming.
// Client Side
// Create a socket object
Socket s = new Socket(host, port);
// Get the output stream from the socket object
OutputStream os = s.getOutputStream();
// Create a file object and read data from it
File f = new File("path/to/file");
FileInputStream fis = new FileInputStream(f); // To read content of file in bytes format
// Read data until there is something to read in byte format // Then write to output stream at server while((longLen=fis.read(byteArray))>0) { os.write(byteArray, 0, longLen); }
// Flush and close output stream os.flush(); os.close();
// Close input and socket stream fis.close(); s.close();
Code language: Java (java)
Click here to try this code yourself
Conclusion
In this guide, we discussed how to transfer files from client to server socket in Java. We discussed the fundamentals of socket programming in Java, including how to create a client and a server, how to bind a socket to a local address and port, and how to send and receive data using a socket. We also discussed how many clients can connect to a server socket and the sockets transfer process. If you are looking for a reliable and efficient way to transfer data from one system to another, socket programming in Java is a great option. With socket programming, you can easily transfer data from one system to another without any hassle.
Frequently Asked Questions to Resolve (FAQs)
How do I transfer files using a socket in Java?
To transfer files using a socket, you will need to establish a connection between the client and server using a socket on each end. Once the connection is established, you can use the send()
and recv()
methods to send and receive data over the socket.
How do you send data from client to server in socket programming?
To send data from a client to a server using a socket, you can use the send() method on the client side and the recv()
method on the server side.
How will you achieve transfer files between the client and server using socket in Java?
To transfer a file between a client and a server using Java sockets, you will need to establish a connection between the client and server using a socket on each end. Once the connection is established, you can use the input and output streams of the socket to send and receive data.
Can a socket be both client and server?
A socket can be both a client and a server
How many clients can connect to a server socket in Java?
The number of clients that can connect to a server socket depends on the server’s resources and configuration. There is no inherent limit to the number of clients that can connect to a server socket. However, there may be practical limits based on the server’s hardware and software capabilities. For example, a server with limited memory and processing power may not be able to handle a large number of concurrent connections. Additionally, the server’s operating system may impose limits on the number of connections that can be made to a socket.
Sharing is caring
Did you like what Divyansh Agrawal wrote? Thank them for their work by sharing it on social media.
No comments so far
Curious about this topic? Continue your journey with these coding courses: